FluentAssertions for Unity
For my current game I'm developing with a friend, I started testing the core engine features from day one using the Unity Test Runner & Assertion Library. To be honest, I dislike the built-in functionality of writing asserts.
They are hard to read and in case of an error, they often don't tell much themselves without adding some more explanation. For example, if you have a code like this:
[Test]
public void MoneyMultiplierWillBeIncreasedWhenLevelingTheModifier()
{
var generator = new FakeGenerator();
generator.ModifierCollection.Add(new FakeModifier());
generator.ModifierCollection.Add(new FakeModifier());
generator.ModifierCollection[0].Build(); // first built modifier does not have a multiplier
generator.ModifierCollection[1].Build(); // first built modifier does not have a multiplier
Assert.IsTrue(Math.Abs(generator.MoneyModifier - 1) < 0.1f, "generator.MoneyModifier == 1");
generator.ModifierCollection[0].Build();
Assert.IsTrue(Math.Abs(generator.MoneyModifier - 1.2f) < 0.1f, $"generator.MoneyModifier == 1.2f, is {generator.MoneyModifier}");
generator.ModifierCollection[1].Build();
Assert.IsTrue(Math.Abs(generator.MoneyModifier - 1.44f) < 0.1f, $"generator.MoneyModifier == 1.44f, is {generator.MoneyModifier}");
generator.ModifierCollection[0].Build();
Assert.IsTrue(Math.Abs(generator.MoneyModifier - 1.728f) < 0.1f, $"generator.MoneyModifier == 1.728f, is {generator.MoneyModifier}");
generator.ModifierCollection[1].Build();
Assert.IsTrue(Math.Abs(generator.MoneyModifier - 2.0736f) < 0.1f, $"generator.MoneyModifier == 2.0736f, is {generator.MoneyModifier}");
}
When executing the test and something is going wrong, then the message will be that one:
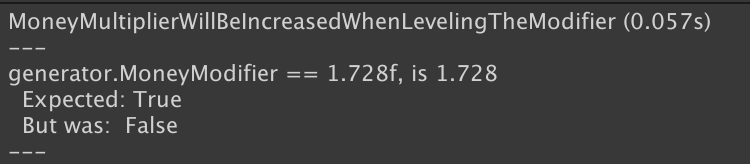
In this case, several things could be true
- I've changed one of the equations leading to a not expected output.
- I've changed the test and forgot to update the text
- Expected: True, But was: False is not helpful at all
That's why I wanted to use FluentAssertions which is a wonderful library for creating readable and maintainable assertions. With them, the code looks like this:
[Test]
public void MoneyMultiplierWillBeIncreasedWhenLevelingTheModifier()
{
var generator = new FakeGenerator();
generator.ModifierCollection.Add(new FakeModifier());
generator.ModifierCollection.Add(new FakeModifier());
generator.ModifierCollection[0].Build(); // first built modifier does not have a multiplier
generator.ModifierCollection[1].Build(); // first built modifier does not have a multiplier
Math.Abs(generator.MoneyModifier).Should().BeApproximately(1, 0.1f);
generator.ModifierCollection[0].Build();
Math.Abs(generator.MoneyModifier).Should().BeApproximately(1.2f, 0.1f);
generator.ModifierCollection[1].Build();
Math.Abs(generator.MoneyModifier).Should().BeApproximately(1.44f, 0.1f);
generator.ModifierCollection[0].Build();
Math.Abs(generator.MoneyModifier).Should().BeApproximately(1.728f, 0.1f);
generator.ModifierCollection[1].Build();
Math.Abs(generator.MoneyModifier).Should().BeApproximately(2.0736f, 0.1f);
}
That looks way better. Cleaner to read, no manually written strings anymore. And in case something is wrong, the following message is reported:

Now it is absolutely clear, what exactly went wrong.
However, using the lib has one bad thing: the context menu with "Open error line" will not work anymore and jumps into the Test Framework instead of the actual test code. I've asked in the Unity Forums, if this could be resolved somehow.
If you want to use FluentAssertions yourself, you can grab the code on GitHub or the Asset Store (currently in review).